How to Fix ASP.NET Core Bugs in A Production Environment
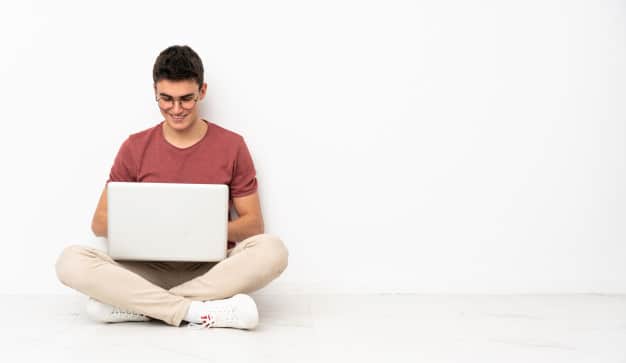
ASP.NET Core bugs in a production environment can be prevented through the use of testing. This can be done with automated tests, such as unit testing. In addition, manual testing can be done through the use of QA engineer.
Whichever way we do it, the end goal is to eliminate production environment bugs.
However, despite all the testing and the process that are in place, there are always some problems that get through the net.
We are going to have a look at some of the ways of how we can detect and fix these bugs.
Logging provider
ASP.NET Core provides a logging provider which can be installed from a Nuget package. The package provides a ILoggerFactory
interface that allows us to create a logger provider.
The types of logging provider is dependant on how we want to write logs. We could write log files to a text file. Alternatively, we could output logs to a third-party monitoring software, such as Sentry.
Whichever logging provider we use, we can customise what logs get outputted to a certain provider. So we may wish to log all outputs to a text file regardless of their level. Whereas, we may only want to log exceptions to our third-party monitoring software.
The logging provider takes advantage of the ILogger
interface. The ILogger
interface includes methods and extension methods that allows us log different components of an application, with different severity levels.
This means we have a number of options of tracking ASP.NET Core production bugs. It is essential that we have some way of logging and alerting for exceptions in an ASP.NET Core production environment. This will need to include the error’s stack trace so we know which part of the application is causing the issue.
Reproduce the problem locally
So we’ve been alerted about a potential defect in our ASP.NET Core production environment. The next step is to fix it. How do we go about doing that?
The first step is to try and recreate it in a local environment. In an ideal world, we would be able to get the same error locally that we would in production. Assuming that is the case, we would implement a fix, go through the usual testing procedures and then launch the fix into production. Job done!
But, what if we can’t recreate it locally? The issue could be down to a specific database record, such as a specific user account. There is a high chance that the local environment database is out-of-sync with the production environment database.
One step is to bring down a copy of the production database to the local environment. That would be ideal if it’s a small database and if we can recreate the problem, it’s job done.
But what other ways can we detect defects?
Performance profiler
Sometimes, it might be difficult to detect where the error is coming from. But, the help of a performance profiler can help pinpoint any potential performance issues.
Visual Studio has a built-in performance issue tool which registers the memory and CPU being used. In-addition, it allows us to take a memory snapshot, so we can see the objects and heap size along with the size for each. It also tells us the duration for these tasks.
We can use VS 2022’s performance profiler by going to Debug > Performance Profiler…. Visual Studio will ask what tools we want to use. Once it’s started, it will load the application and at this point we can take a snapshot so we can recreate the issue.
Once we’ve finished with the performance profiler, it will generate a detailed report for us. This includes the memory and CPU usage, as well as database queries that have been made.
Prevent bugs from happening
Ultimately, it’s better that production environment bugs don’t occur. Proper testing and deployment procedures are essential for this to happen.
Having poor procedures can lead to more man hours being spent fixing in the problems in the long run. And these issues can cause self-doubt in the application’s performance and reliability.
So it’s always best to go above and beyond when it comes to testing.